
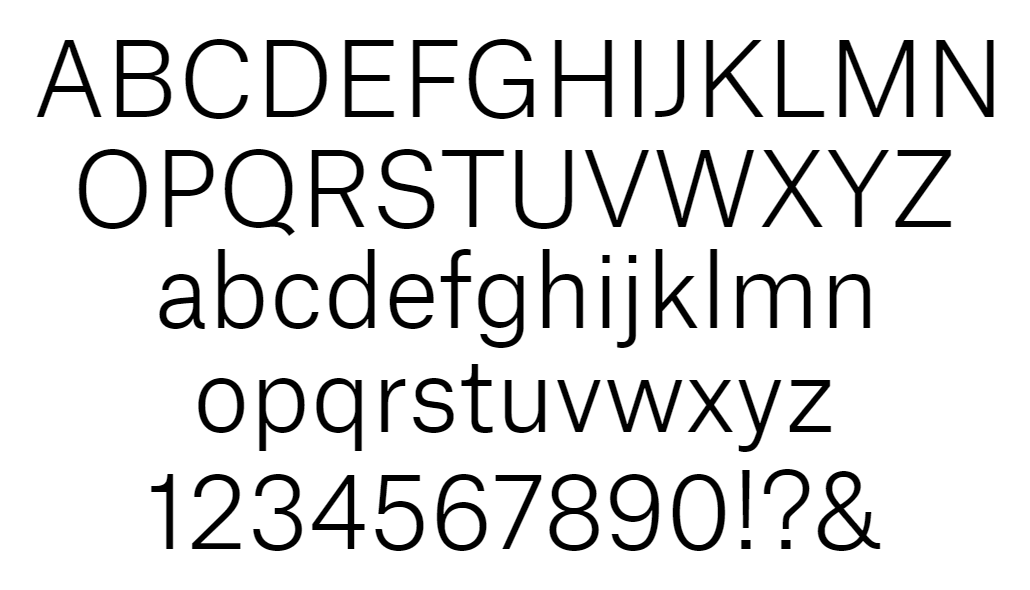
Notice how we're passing the dest's w and h variables by reference, so the values can be set. It takes as its arguments the font, the character string (in our case, this will be the single character), and variables to hold the width and height. Now for another important bit - we want to find out the dimensions of the glyph we've just created, so we can know if we can place it on the atlas at the desired coordinates. A short SDL_TTF string, composed of a single character. We next call TTF_RenderUTF8_Blended, passing in our one character string (c), the font we're working with, and our pre-defined white colour. The NULL terminator is important for the next step. In effect, this will create a char array holding a single ASCII value, followed by a NULL terminator. For each iteration of the loop, we set the first index of c to the value of i, and the second index to 0. Working from ' ' (a space) to 'z' will see us starting from 32 to 122 in integer values and present all the alphanumeric values we want, along with some symbols and punctuation. We set the dest's x and y to 0,0 to start with, then begin iterating through a subset of the ASCII table. Our dest SDL_Rect will be used to determine the rectangular region we will be drawing the glyph at. With our prep down, we're ready to start drawing our glyphs on the atlas. It will be no good if our text always had an opaque background. The arguments and SDL_SetColorKey will help to ensure that our surface is transparent, which will be important when using the texture.
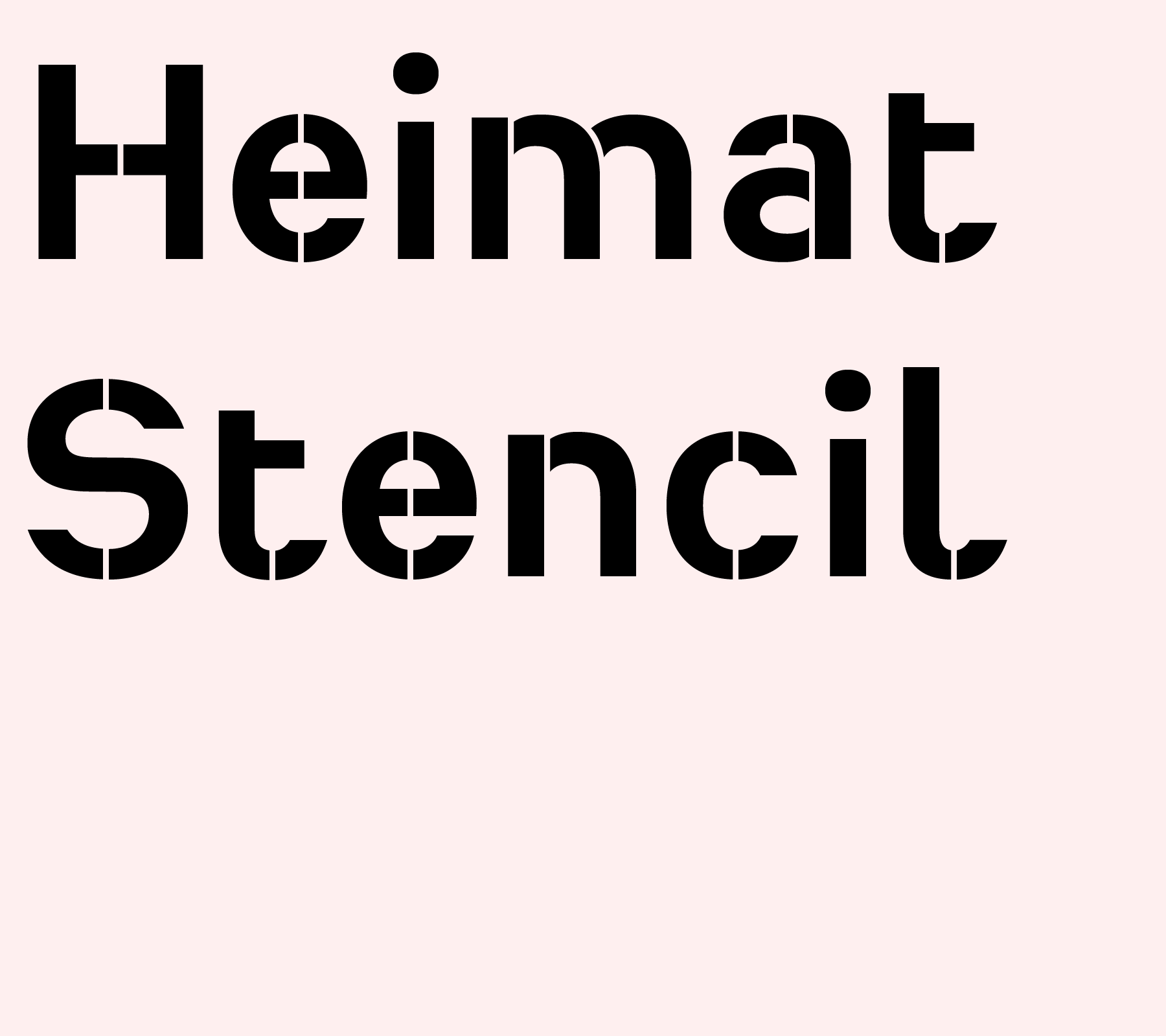
In this case, FONT_TEXTURE_SIZE is 512, resulting in a square power-of-two texture. We create an SDL_Surface of the size of our defined FONT_TEXTURE_SIZE. With that done, we open our file, once again at the index for the font we want to work with, and for the filename argument specified, and our fixed FONT_SIZE define. We do this with the value of NUM_GLYPHS, to marry up with the size of the array. The next thing we want to do is zero the memory for our array of glyths, using memset, for the font we're working with. Notice how the char array is only 2 items wide. We're first setting up a few variables to work with - two SDL_Surfaces, two SDL_Rects, and a char array. SDL_BlitSurface(text, NULL, surface, &dest) įontTextures = toTexture(surface, 1) SDL_LogMessage(SDL_LOG_CATEGORY_APPLICATION, SDL_LOG_PRIORITY_CRITICAL, "Out of glyph space in %dx%d font atlas texture map.", FONT_TEXTURE_SIZE, FONT_TEXTURE_SIZE) If (dest.y + dest.h >= FONT_TEXTURE_SIZE) SDL_SetColorKey(surface, SDL_TRUE, SDL_MapRGBA(surface->format, 0, 0, 0, 0)) Surface = SDL_CreateRGBSurface(0, FONT_TEXTURE_SIZE, FONT_TEXTURE_SIZE, 32, 0, 0, 0, 0xff) Memset(&glyphs, 0, sizeof(SDL_Rect) * NUM_GLYPHS) įonts = TTF_OpenFont(filename, FONT_SIZE) Static void initFont(int fontType, char *filename) Take a look at the listing below and we'll then go through it. The initFont function itself is quite meaty, but is the most important part of this tutorial, as it is where we will set up our font atlas. The initFont function takes the font type (the enum value, which in itself can be expressed as an int), and the filename we want to use. InitFont(FONT_LINUX, "fonts/LinLibertine_DR.ttf") InitFont(FONT_ENTER_COMMAND, "fonts/EnterCommand.ttf") The initFonts now creates the font atlas for both our fonts, by making a call to initFont: We won't be working with all of these, however, just a subset of the printable characters that we'll be working with.
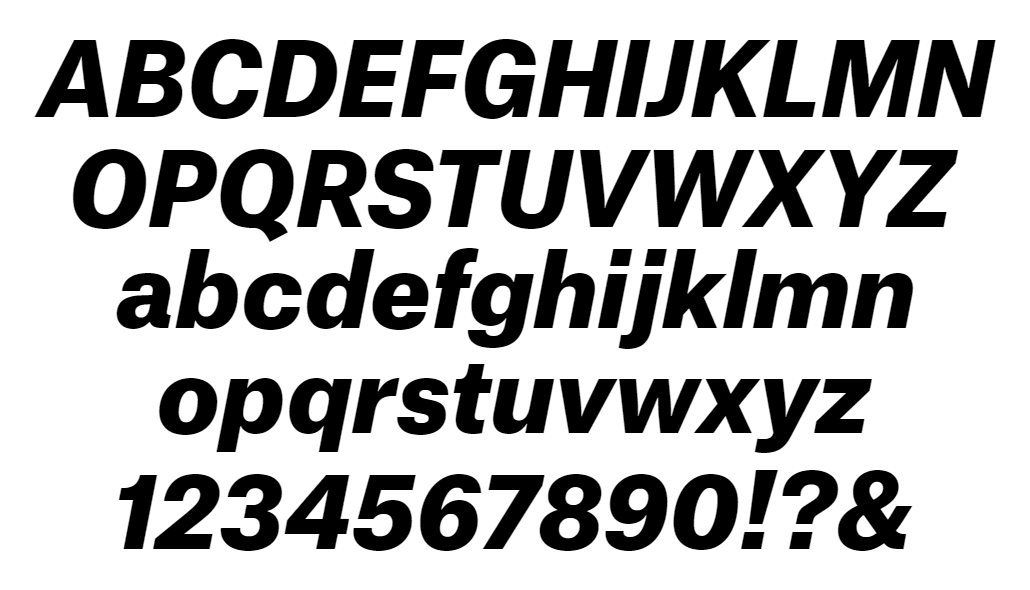
Our glyphs array is 128 entries wide (according to the NUM_GLYPHS define in the header), enough to hold the entire ASCII table. These variables will be used to hold the glyph atlas positions, as well as the font texture we will be holding for each font. We've also introduced two new arrays, glyphs and fontTexture.
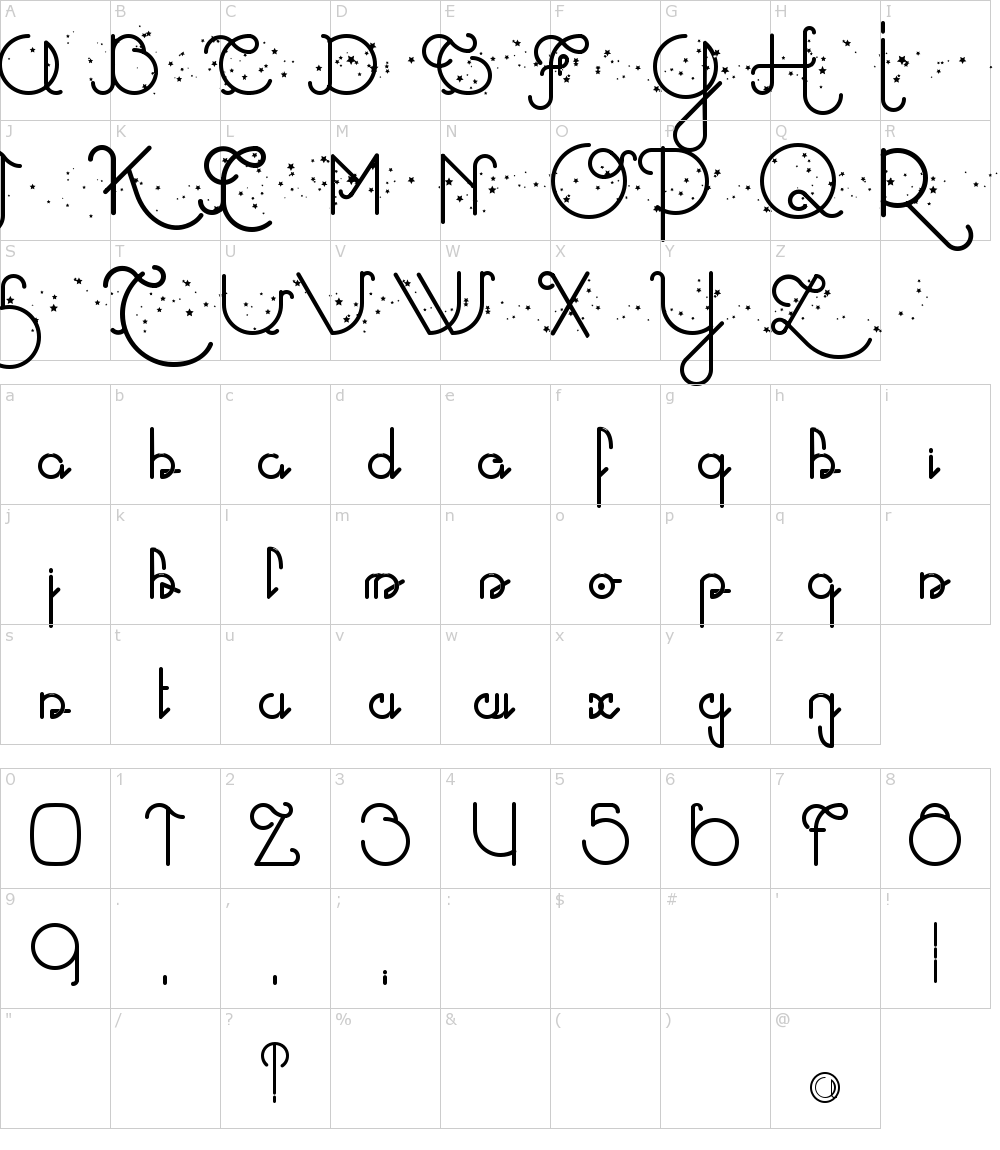
As such, the TTF_FONT static variable is now an array. One of the first things that has changed is that we're now supporting multiple fonts. A quick note: we've added an enum to defs.h to define the fonts we'll be using. We'll start with text.c, as this deals with the actual setup and use of our font atlas. The major changes in this tutorial have been done in text.c and demo.c. You will see a window open like the one above, with a number of lines of text, including two at the bottom that are showing incrementing numbers. It'll be like creating the Sprite Atlas we looked at in a previous tutorial, except without the packing aspect this will be a little simpler.Įxtract the archive, run make, and then use. We'll then pick our the glyphs we want to use to draw our text. In this tutorial, we'll do just that, by creating a single texture onto which we'll place all our glyphs. It would be nice if there was another way to draw our text strings, without needing to create a short-lived texture.
